Implementing a Single Custom Login Page for all Panels in FilamentPHP
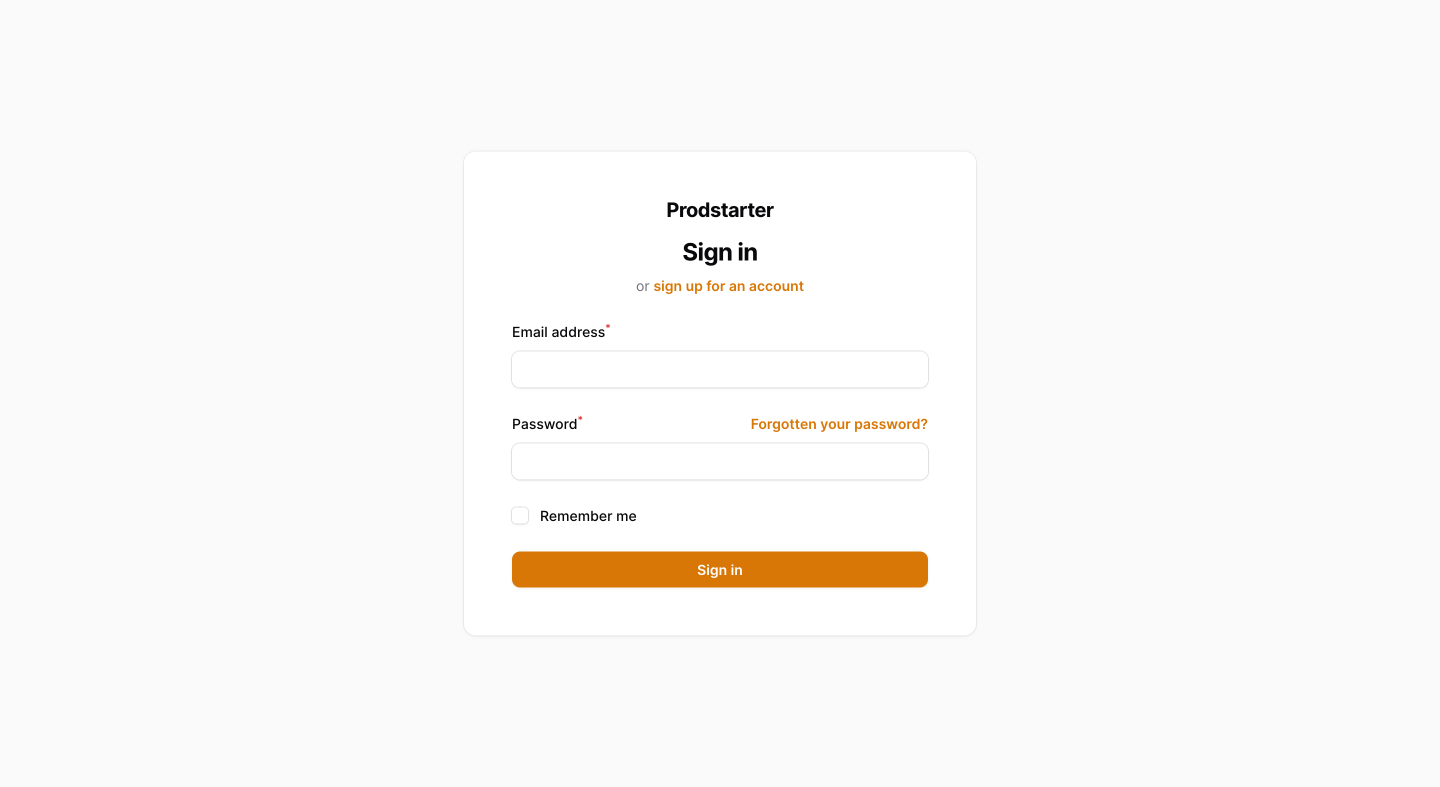
Filament is a robust, open-source admin panel meticulously crafted in PHP and designed to run seamlessly on the widely-used Laravel framework.
Filament simplifies intricate tasks such as constructing responsive admin panels, managing CRUD operations (Create, Read, Update, Delete), and creating tables and other essential elements. This allows developers to channel their focus where it truly matters: their core business logic.
Introducing Panels
Filament's panel structure acts as the primary container, enabling the construction of feature-rich admin panels. These panels encompass a wide array of components, including pages, resources, forms, tables, notifications, actions, infolists, and widgets.
Filament provides the flexibility to create multiple panels, each equipped with a default dashboard that can be customized with widgets offering various capabilities, such as displaying statistics, charts, tables, and more.
To create a new panel, utilize the make:filament-panel command, providing the unique name of the new panel:
php artisan make:filament-panel app
To enable authentication (login) for the panel, add the login method to the panel configuration:
use Filament\Panel; public function panel(Panel $panel): Panel { return $panel // ... ->path('app') ->login(); }
Assuming you're building a Filament app for different user types, with each user type having its panel, you can create a single custom authentication page for all users and redirect them to their respective panels after login. Here's how
1. Create an Auth Panel dedicated to handling authentication, such as login.
php artisan make:filament-panel auth
In the AuthPanelProvider configuration, add the ->login() and ->registration() methods:
class AuthPanelProvider extends PanelProvider { public function panel(Panel $panel): Panel { return $panel ->default() ->id('auth') ->path('auth') ->login() ->registration() ->passwordReset(); } }
2. Create a middleware to redirect users if not authenticated.
After adding the login and registration methods to the auth panel, create a new middleware in the Laravel Middleware folder, such as RedirectIfNotFilamentAuthenticated. Modify the authMiddleware configuration section of the AuthPanelProvider class:
<?php namespace App\Http\Middleware; use Filament\Facades\Filament; use Filament\Http\Middleware\Authenticate as Middleware; class RedirectIfNotFilamentAuthenticated extends Middleware { protected function redirectTo($request): ?string { return route('filament.auth.auth.login'); } }
Replace the authMiddleware config section of the AuthPanelProvider class with the new middleware:
Original config:
->authMiddleware([ Authenticate::class, ])
New Config:
->authMiddleware([ RedirectIfNotFilamentAuthenticated::class, ])
Update this configuration for every other panel you've created or will create, replacing the section with the new middleware.
Limited-time offer - $350/Week
Turn your vision into reality. Our subscription service offers continuous Laravel Filament development and support. Start building your future today!
Related Articles that May Be to Your Interest
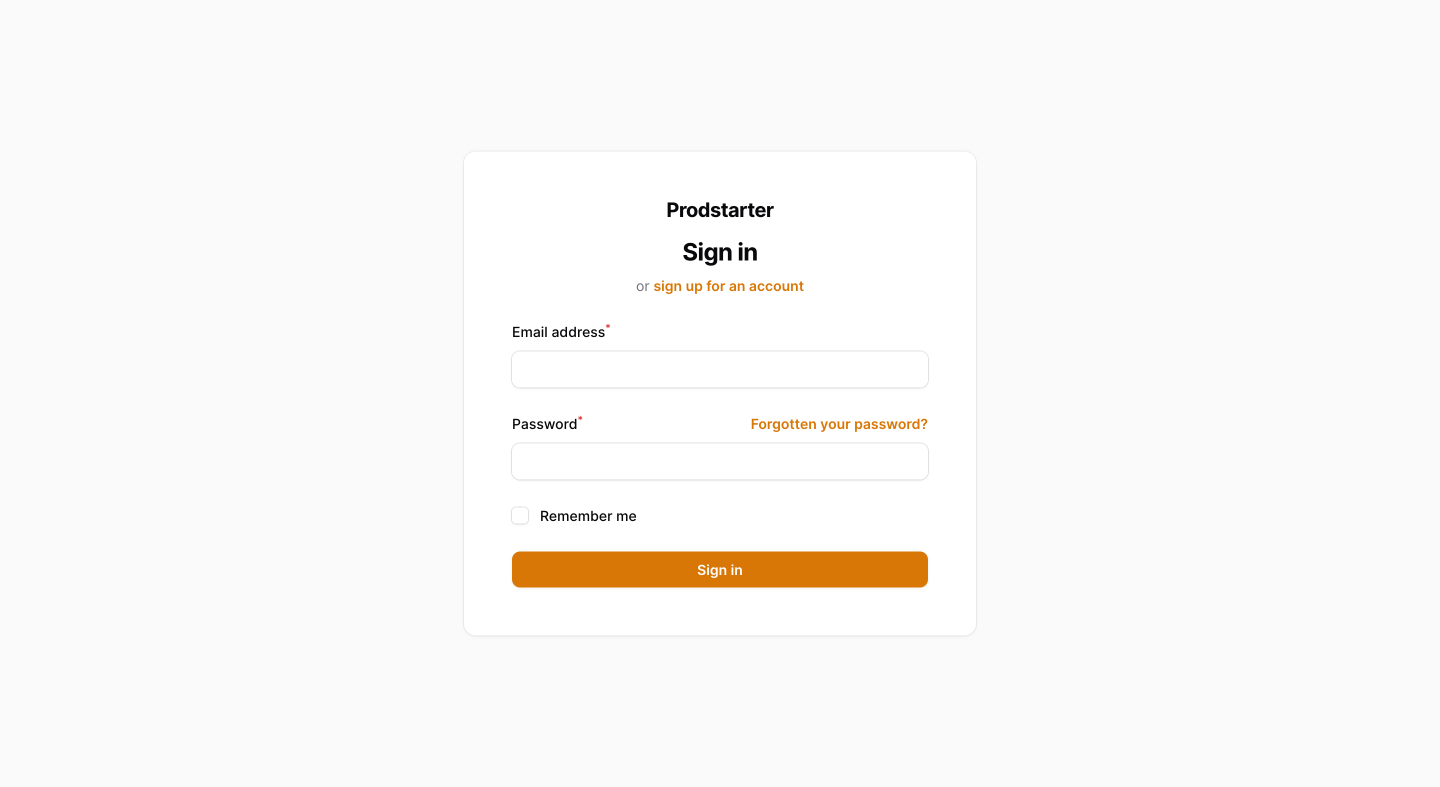
Implementing a Single Custom Login Page for all Panels in FilamentPHP
Learn how to use custom page for login and registration across multiple panels in FilamentPHP.
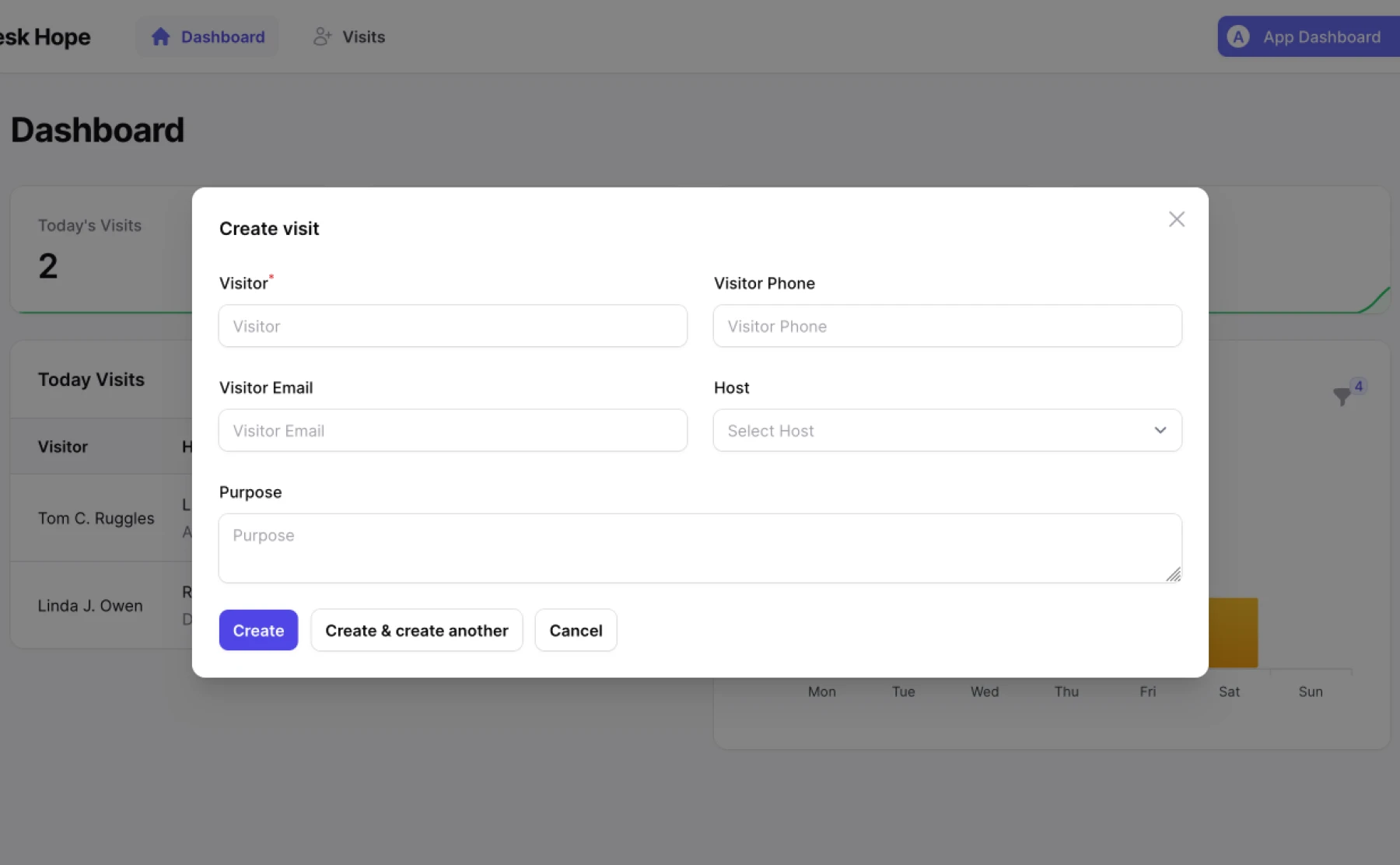
Introducing FrontDesk: Your Open-Source Visitor Management System
Streamline visitor check-ins with FrontDesk, an open-source Visitor Management System built with Laravel & Filament PHP.
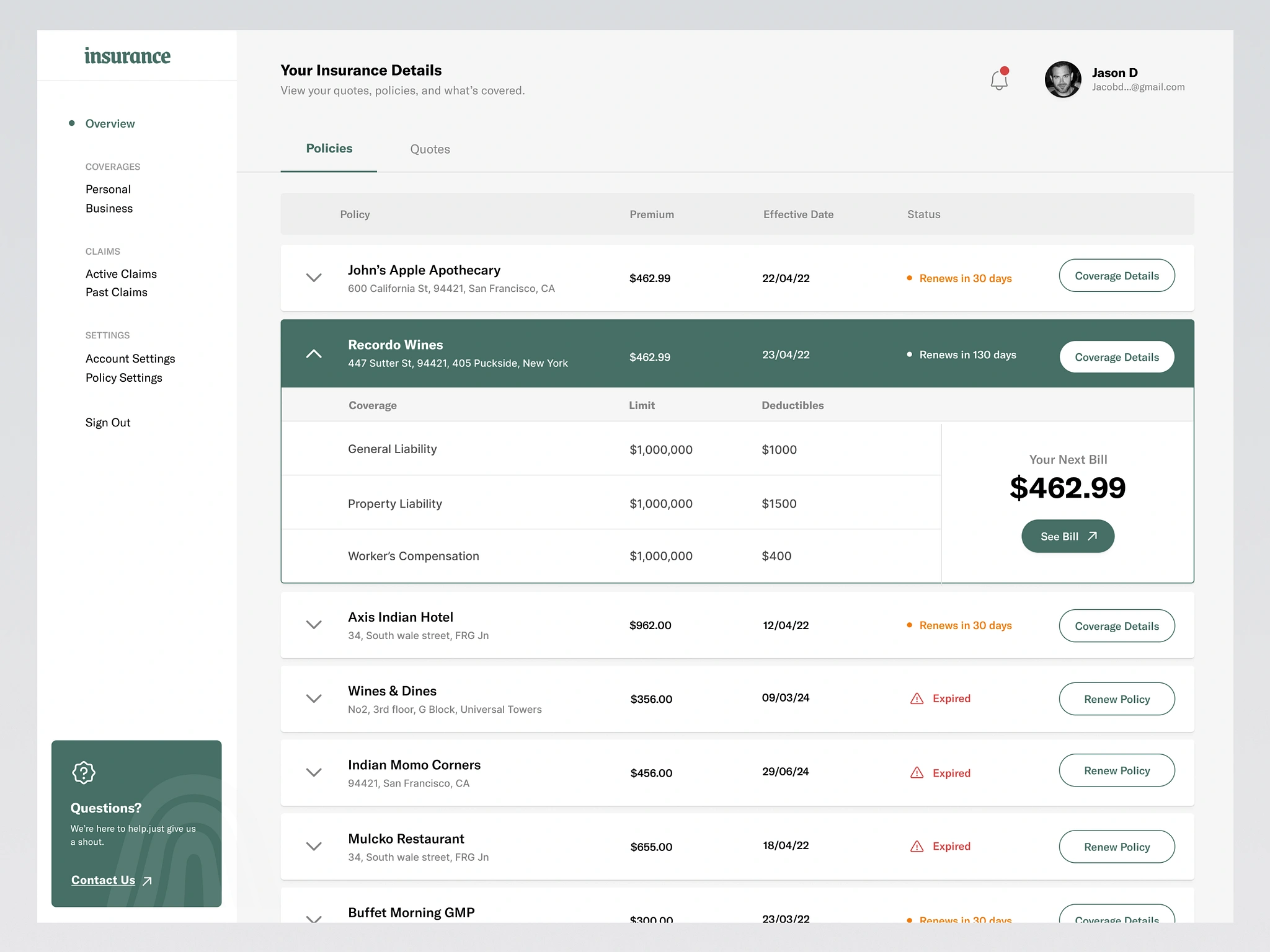
From Data Overload to Clarity: Why Your Business Needs a Custom Dashboard
Custom dashboards streamline decision-making with predictive analytics, interactive visuals, and smart alerts.